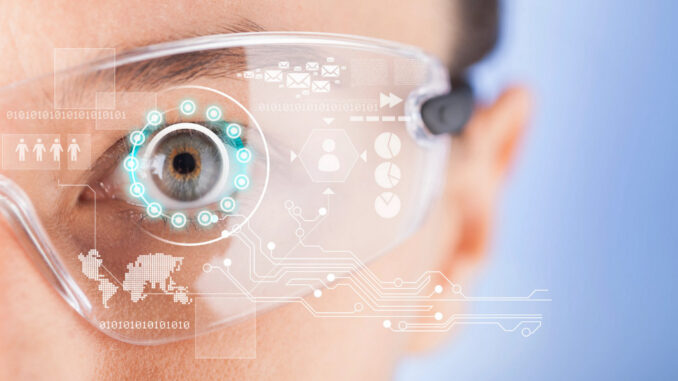
Learning programming structures is crucial for developing strong problem-solving skills and understanding how to write efficient and maintainable code.
Here are the key programming structures you should focus on, along with resources to help you learn them:
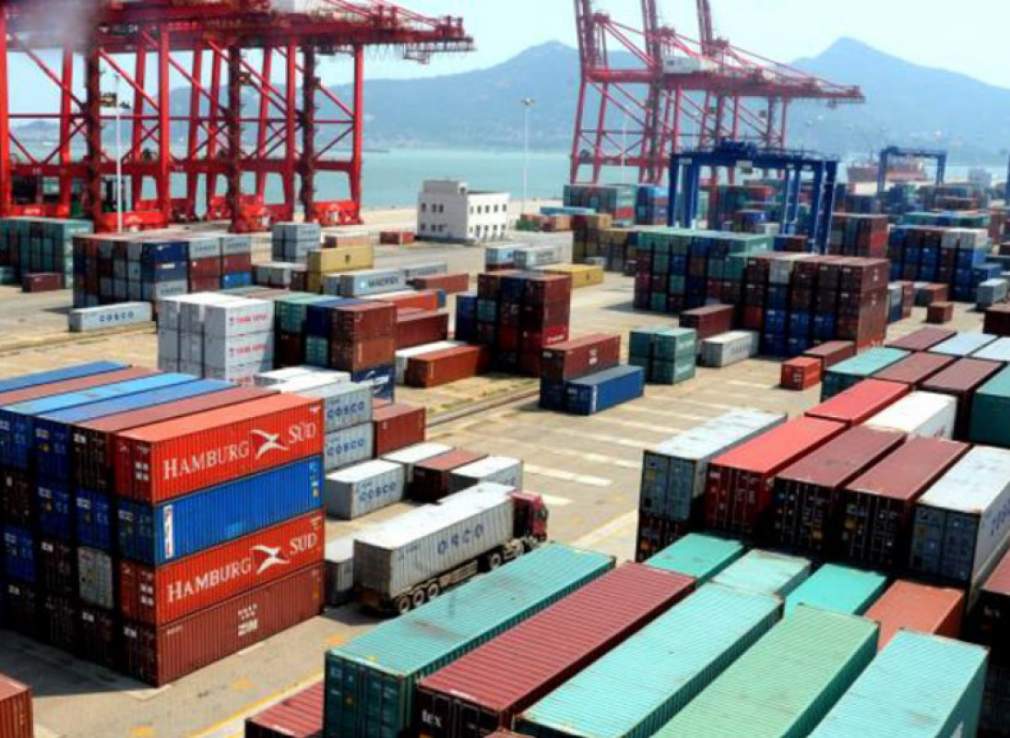
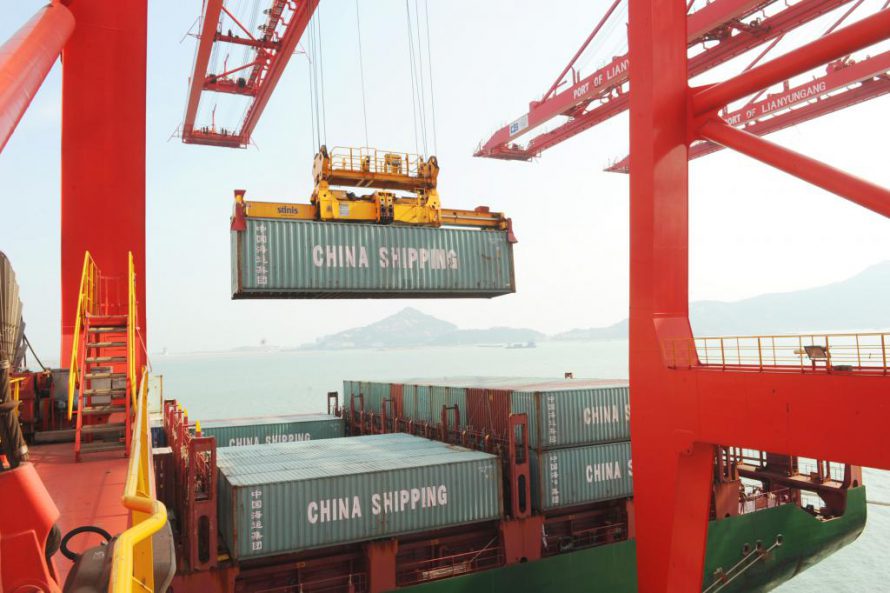
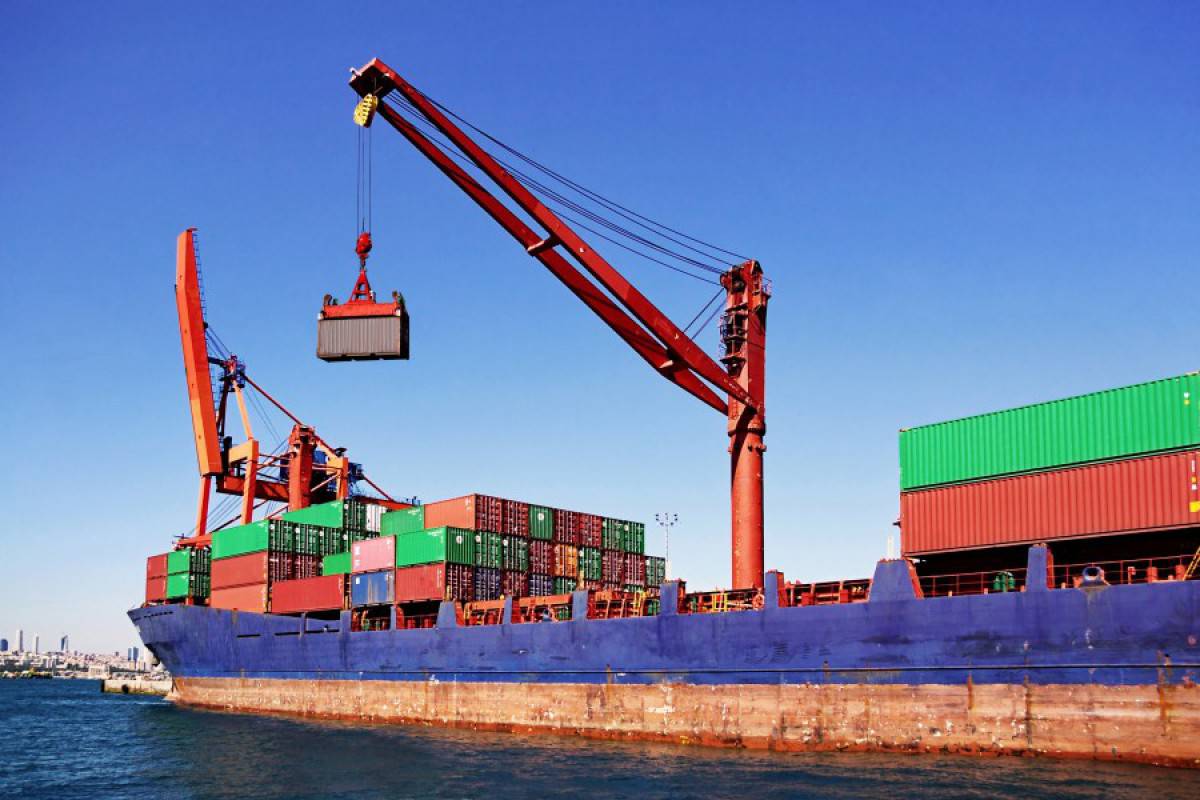
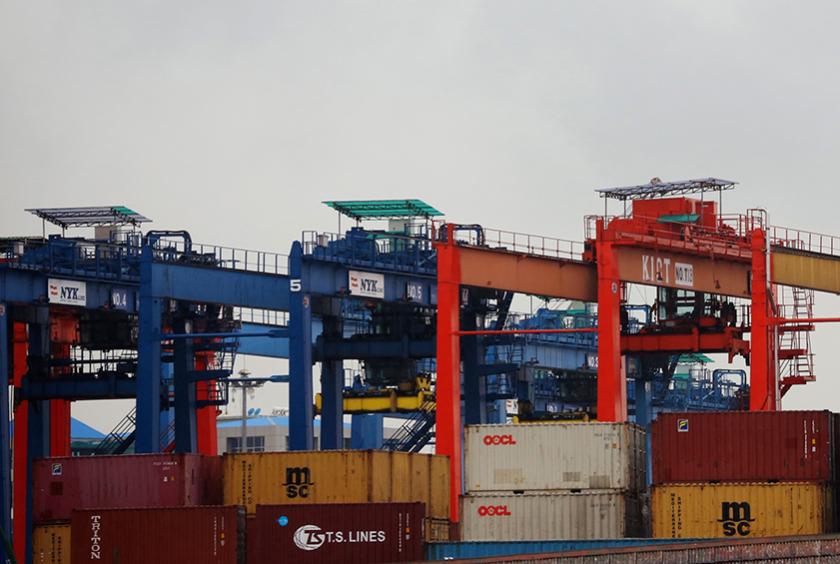
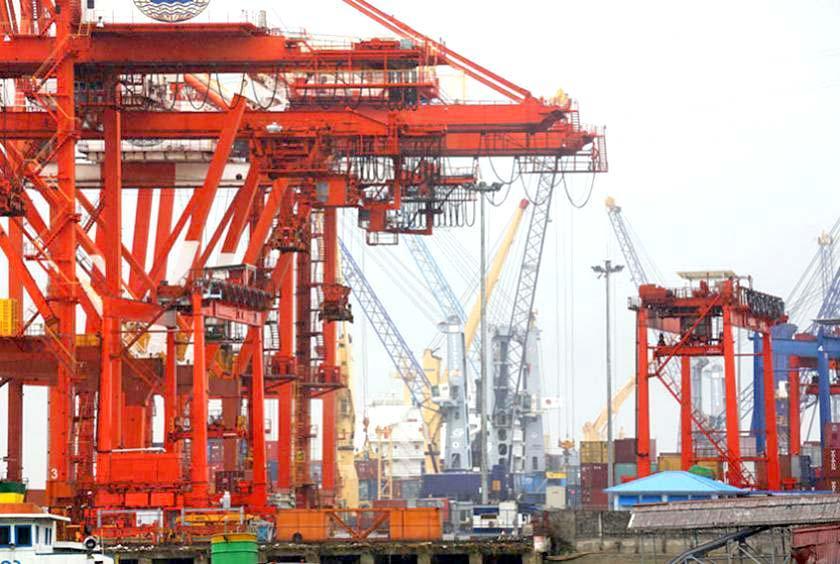
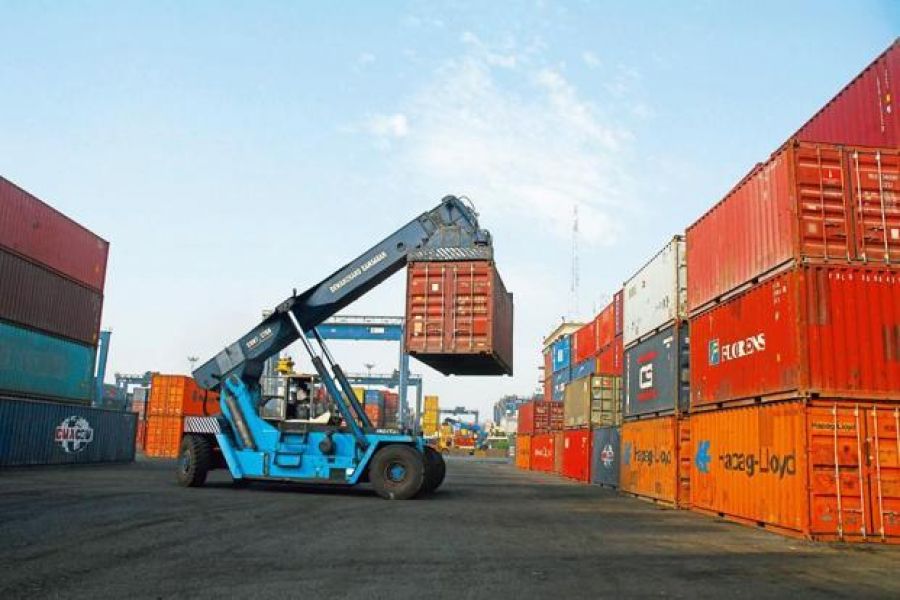
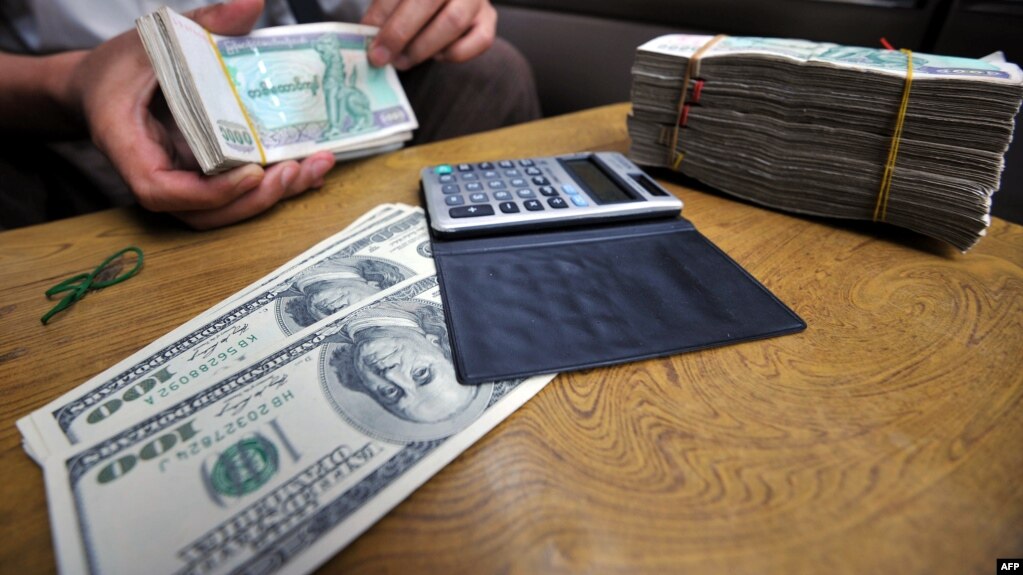
1. Data Structures
Understanding how data is organized, managed, and stored is fundamental to efficient programming.
Arrays and Lists: Basic data structures for storing collections of elements.
Linked Lists: Structures where elements point to the next element, allowing for efficient insertion and deletion.
Stacks and Queues: Abstract data types for managing elements in a last-in-first-out (LIFO) and first-in-first-out (FIFO) manner, respectively.
Trees: Hierarchical data structures with nodes connected by edges. Special types include binary trees, AVL trees, and B-trees.
Graphs: Data structures consisting of nodes (vertices) and edges, used to represent networks.
Hash Tables: Structures that map keys to values for efficient data retrieval.
Resources:
“Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
“Algorithms, Part I” and “Algorithms, Part II” on Coursera by Princeton University
GeeksforGeeks Data Structures tutorials
2. Algorithms
Algorithms are step-by-step procedures for calculations and data processing.
Sorting Algorithms: Bubble sort, selection sort, insertion sort, merge sort, quicksort, and heapsort.
Searching Algorithms: Linear search, binary search, and depth-first search (DFS) and breadth-first search (BFS) for graphs.
Dynamic Programming: Techniques for solving complex problems by breaking them down into simpler subproblems.
Greedy Algorithms: Algorithms that make locally optimal choices at each step.
Divide and Conquer: Techniques that divide the problem into smaller parts, solve each part, and combine the results.
Resources:
“Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
“The Algorithm Design Manual” by Steven S. Skiena
Coursera’s “Algorithm Design and Analysis” course
3. Object-Oriented Programming (OOP)
OOP is a programming paradigm based on the concept of objects, which can contain data and methods.
Classes and Objects: The basic building blocks of OOP.
Inheritance: Mechanism to create new classes from existing ones.
Polymorphism: Ability to process objects differently based on their data type or class.
Encapsulation: Bundling the data with the methods that operate on the data.
Abstraction: Hiding complex implementation details and showing only the necessary features.
Resources:
“Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides
“Head First Design Patterns” by Eric Freeman and Elisabeth Robson
Coursera’s “Object-Oriented Programming in Java Specialization” by UC San Diego
4. Functional Programming
Functional programming is a paradigm where programs are constructed by applying and composing functions.
Pure Functions: Functions that have no side effects and return the same output for the same input.
First-Class Functions: Treating functions as first-class citizens, meaning they can be passed as arguments, returned from other functions, and assigned to variables.
Higher-Order Functions: Functions that take other functions as arguments or return them as results.
Immutability: Data structures that cannot be modified after creation.
Recursion: Functions that call themselves to solve smaller instances of the problem.
Resources:
“Functional Programming in Scala” by Paul Chiusano and Rúnar Bjarnason
“Structure and Interpretation of Computer Programs” by Harold Abelson and Gerald Jay Sussman
Coursera’s “Functional Programming in Scala” by École Polytechnique Fédérale de Lausanne
5. Concurrency and Parallelism
These concepts are essential for writing programs that perform multiple tasks simultaneously.
Threads and Processes: Basic units of execution in concurrent programming.
Synchronization: Mechanisms to ensure that multiple threads or processes can operate safely on shared data.
Locks and Semaphores: Tools for managing access to shared resources.
Concurrent Data Structures: Data structures designed to be safely used by multiple threads simultaneously.
Parallel Algorithms: Algorithms that divide tasks into subtasks that can be executed in parallel.
Resources:
“Java Concurrency in Practice” by Brian Goetz
“Concurrency in C# Cookbook” by Stephen Cleary
Coursera’s “Parallel Programming” by Rice University
6. Design Patterns
Design patterns are typical solutions to common problems in software design.
Creational Patterns: Singleton, Factory, Builder, Prototype.
Structural Patterns: Adapter, Composite, Proxy, Flyweight.
Behavioral Patterns: Strategy, Observer, Command, State.
Resources:
“Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides
“Head First Design Patterns” by Eric Freeman and Elisabeth Robson
Refactoring.Guru Design Patterns website
7. Software Engineering Principles
Understanding software engineering principles is essential for writing maintainable and scalable code.
SOLID Principles: Five principles of object-oriented design for writing understandable and flexible software.
DRY (Don’t Repeat Yourself): Principle of reducing the repetition of code.
KISS (Keep It Simple, Stupid): Principle of simplicity in design.
YAGNI (You Aren’t Gonna Need It): Principle of avoiding over-engineering.
TDD (Test-Driven Development): Writing tests before code to ensure functionality.
Resources:
“Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin
“The Pragmatic Programmer: Your Journey to Mastery” by Andrew Hunt and David Thomas
Coursera’s “Software Engineering Specialization” by University of Alberta
By focusing on these programming structures and principles, you will gain a deep understanding of how to design and implement efficient, maintainable, and scalable software, regardless of the programming language you use.
Leave a Reply