Input validation and sanitization are critical security measures used to prevent a wide range of vulnerabilities in web applications, including Cross-Site Scripting (XSS), SQL injection, and other types of code injection attacks.
Client-Side Validation: Implement validation logic on the client-side using JavaScript to ensure that user input meets specified criteria (e.g., required fields, valid email format).
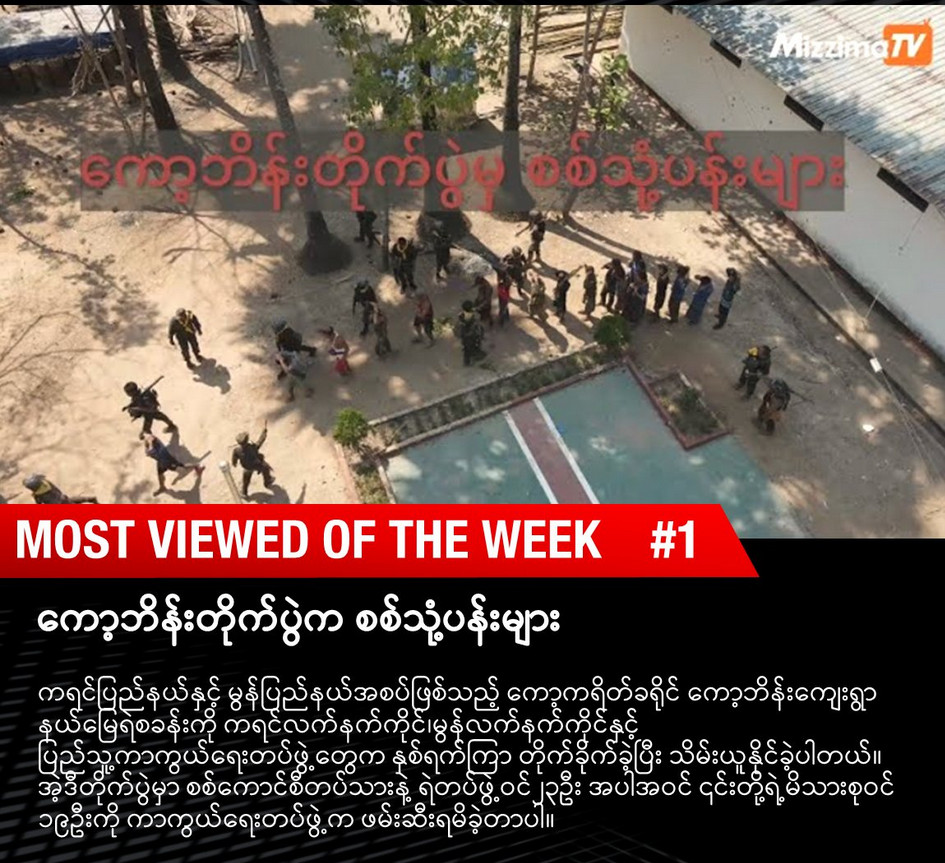
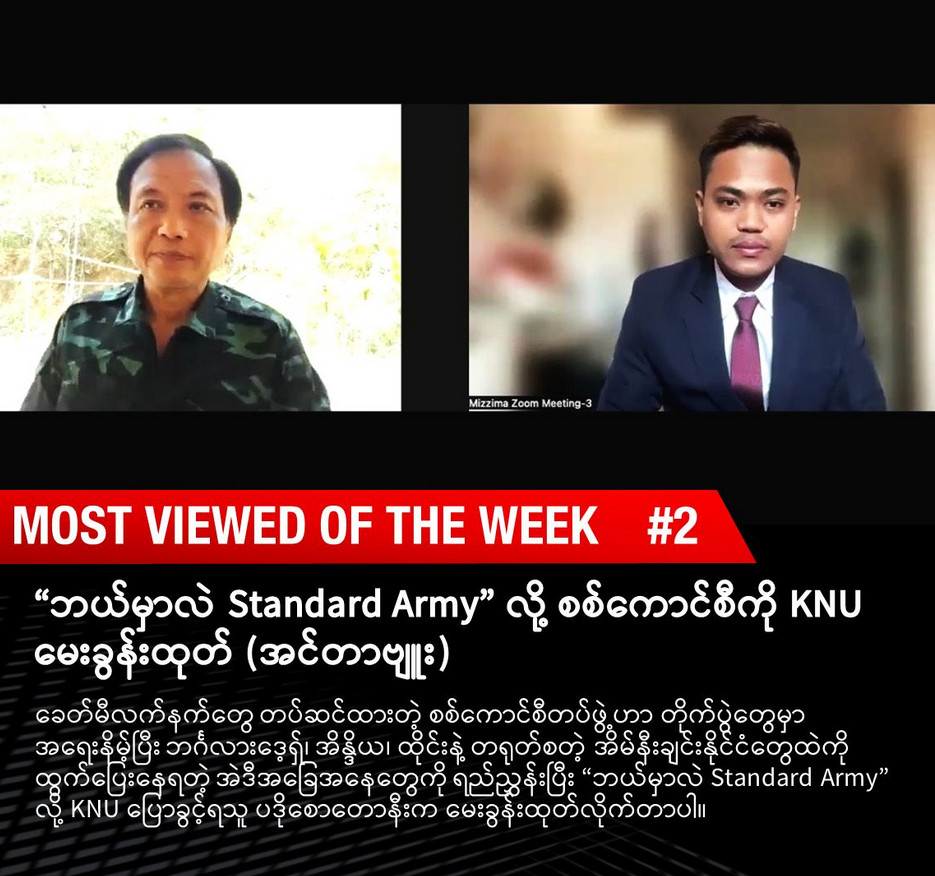
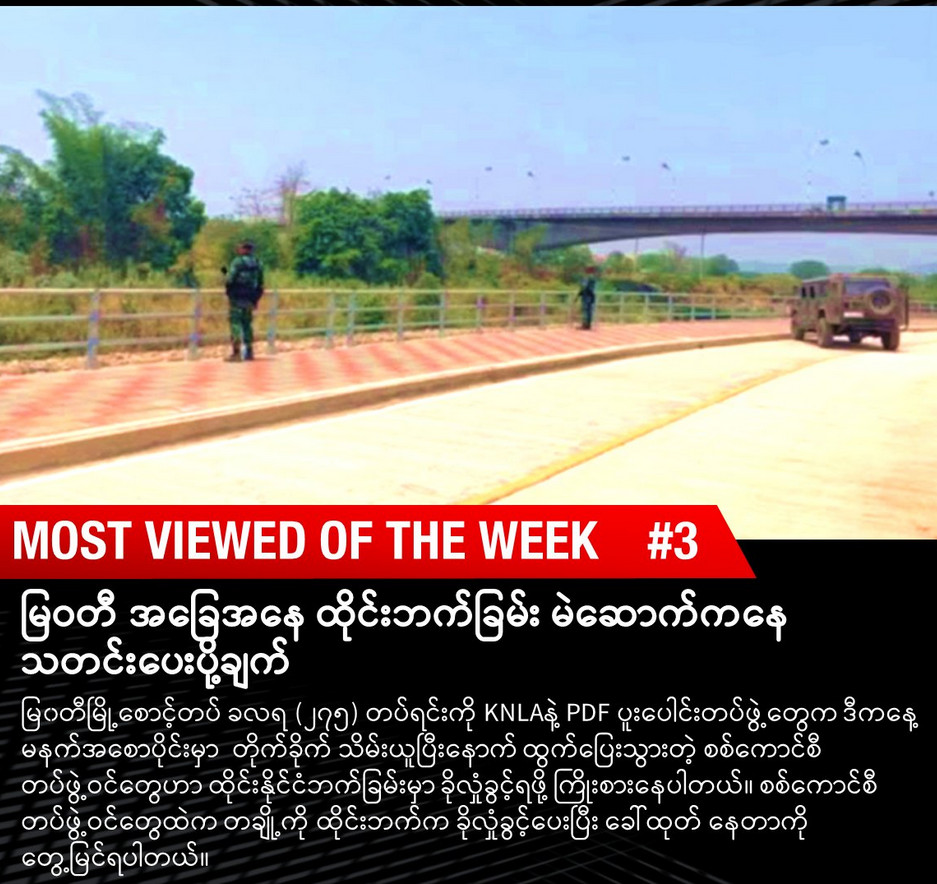
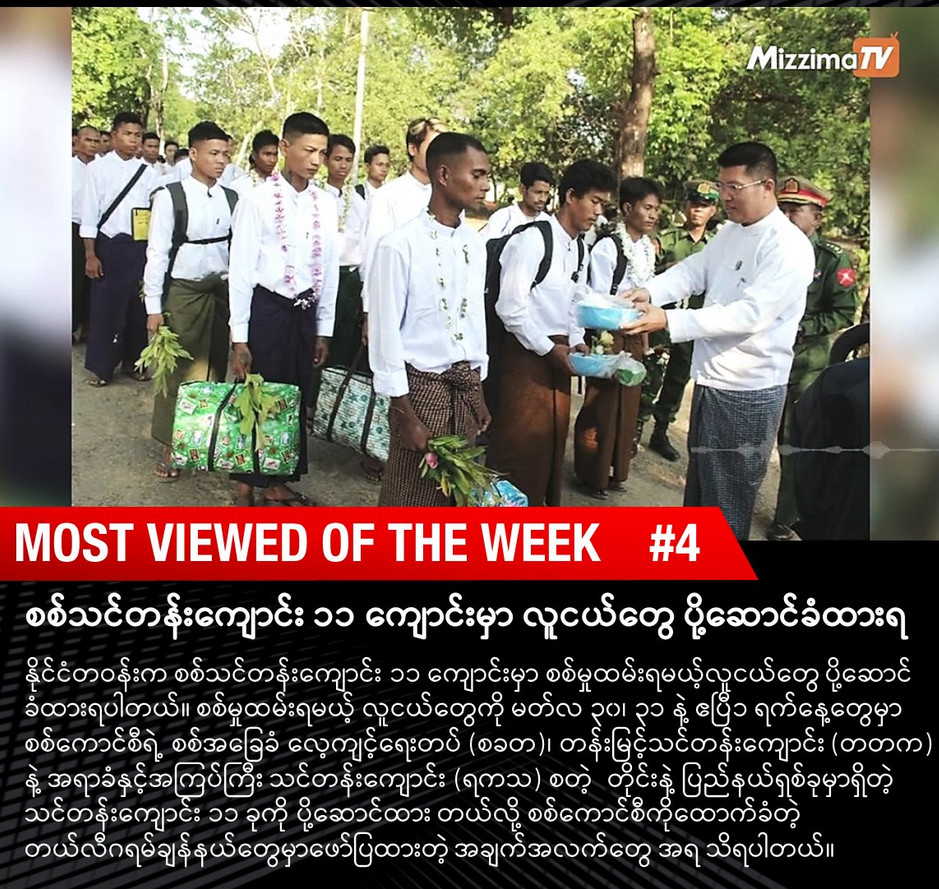
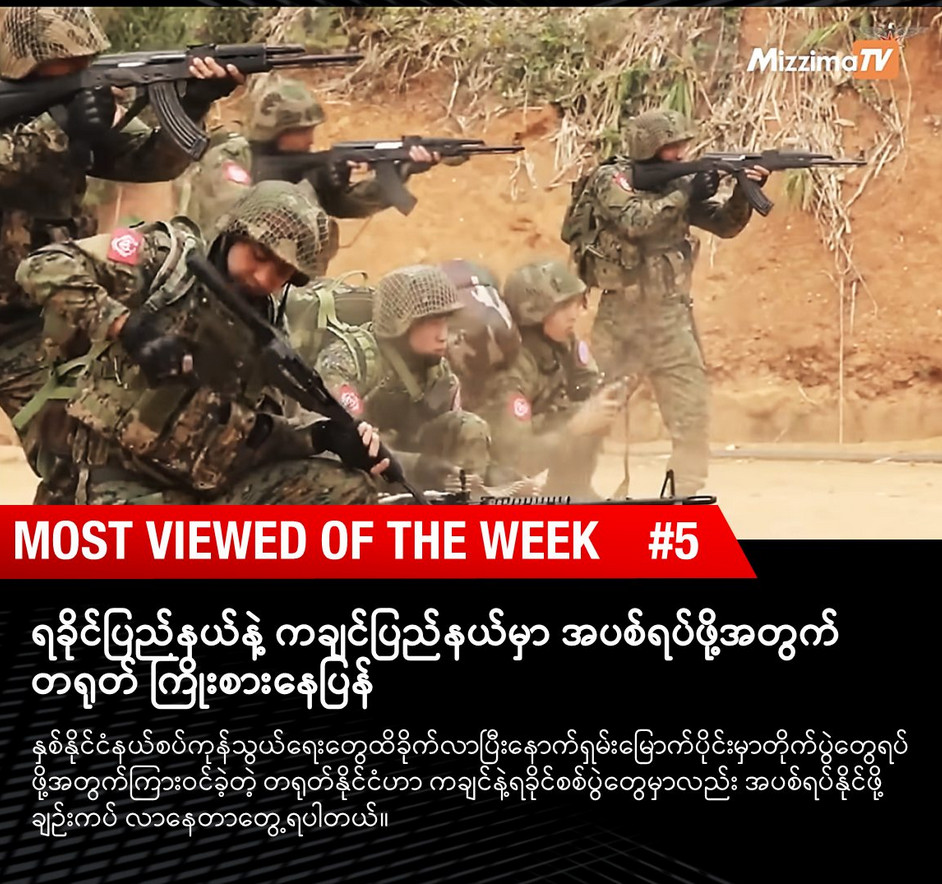
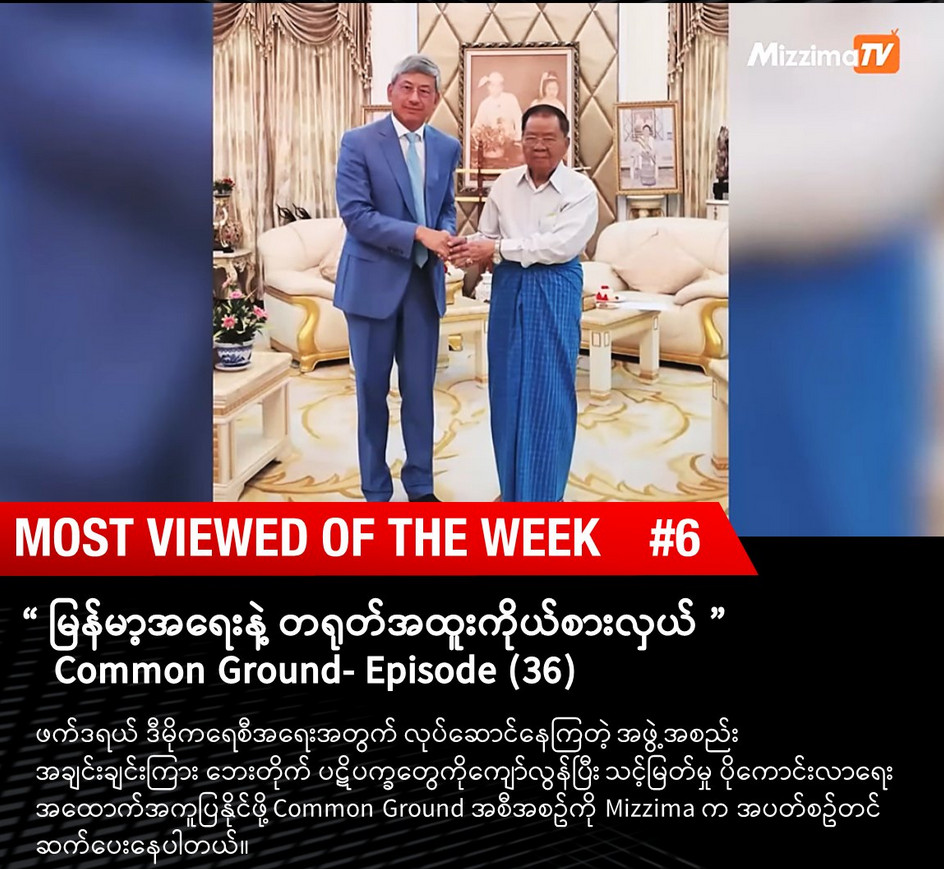
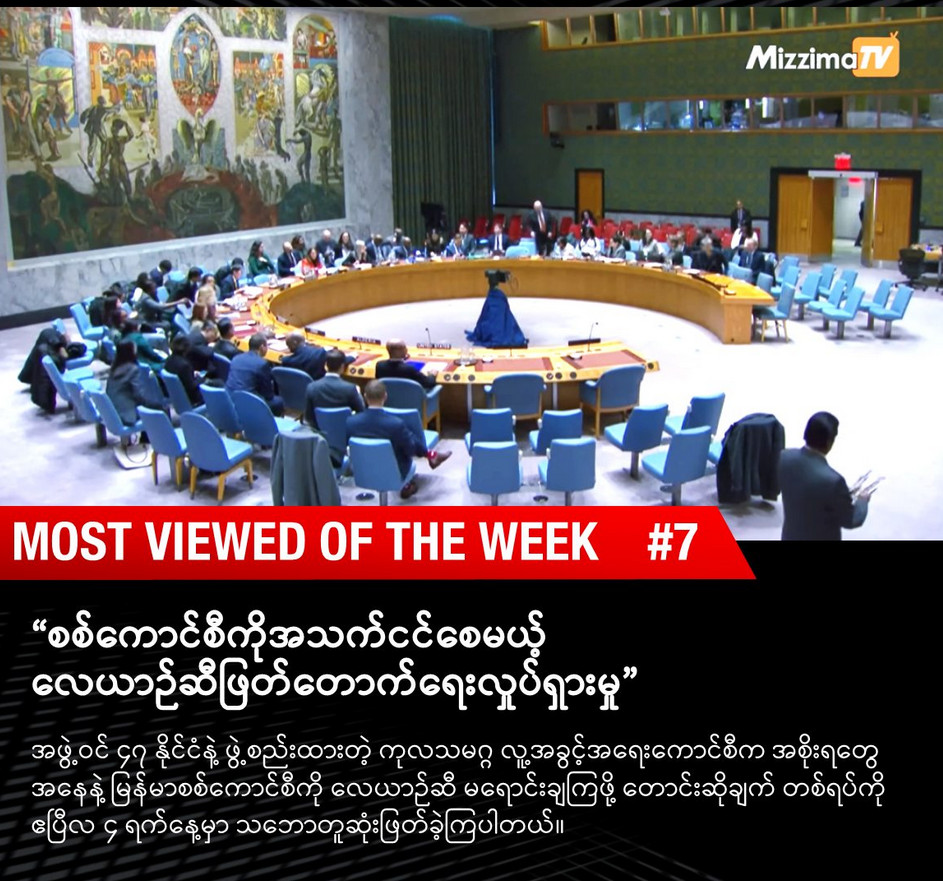
While client-side validation can enhance user experience by providing immediate feedback, it should always be supplemented by server-side validation.
Server-Side Validation: Perform validation checks on the server-side to verify the integrity and correctness of user input. This includes checking data types, length limits, format constraints, and business rules. Server-side validation is crucial as client-side validation can be bypassed by attackers.
Sanitization:
HTML Sanitization: For user-generated content that will be displayed in HTML documents (e.g., comments, forum posts), HTML sanitization is essential to prevent XSS attacks. Use libraries or frameworks that provide HTML sanitization functionality to strip out or escape potentially dangerous HTML tags and attributes.
SQL Sanitization (Parameterized Queries): When constructing SQL queries dynamically, use parameterized queries (prepared statements) instead of concatenating user input directly into SQL queries. Parameterized queries separate SQL code from user input, preventing SQL injection attacks by treating user input as data rather than executable code.
Validation Libraries and Frameworks:
Utilize validation libraries and frameworks provided by your programming language or web development framework. These libraries offer pre-built validation rules, sanitization functions, and mechanisms for handling user input securely. Examples include Laravel Validation for PHP, Express Validator for Node.js, and Django Forms for Python.
Regular Expression (RegEx):
Regular expressions are powerful tools for validating and sanitizing text input based on patterns. Use regular expressions to enforce specific formats (e.g., email addresses, phone numbers) and to filter out or replace unwanted characters.
Whitelist Approach:
Adopt a whitelist approach when validating and sanitizing input. Define a set of acceptable characters, patterns, or formats for each input field, and reject any input that does not match these criteria. Whitelisting is more effective and secure than blacklisting, as it focuses on allowing only known safe inputs.
Error Handling:
Implement comprehensive error handling mechanisms to provide meaningful error messages to users when validation or sanitization fails. Avoid disclosing sensitive information in error messages that could be exploited by attackers.
By incorporating input validation and sanitization into your web application’s development process, you can significantly reduce the risk of security vulnerabilities and protect your application and users from malicious attacks.
Leave a Reply